The Connect class connects to a web page using Basic authentication. It takes a name and a password and concatenates them with a colon in between. It Base64 encodes the resulting string. It makes a URL connection to a web site and sets the 'Authorization' request property to be 'Basic <base-64-encoded-auth-string>' . It reads the content from the URL and displays it to standard output.
Connect.java
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import org.apache.commons.codec.binary.Base64;
public class Connect {
public static void main(String[] args) {
try {
String webPage = "http://192.168.1.1";
String name = "admin";
String password = "admin";
String authString = name + ":" + password;
System.out.println("auth string: " + authString);
byte[] authEncBytes = Base64.encodeBase64(authString.getBytes());
String authStringEnc = new String(authEncBytes);
System.out.println("Base64 encoded auth string: " + authStringEnc);
URL url = new URL(webPage);
URLConnection urlConnection = url.openConnection();
urlConnection.setRequestProperty("Authorization", "Basic " + authStringEnc);
InputStream is = urlConnection.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
int numCharsRead;
char[] charArray = new char[1024];
StringBuffer sb = new StringBuffer();
while ((numCharsRead = isr.read(charArray)) > 0) {
sb.append(charArray, 0, numCharsRead);
}
String result = sb.toString();
System.out.println("*** BEGIN ***");
System.out.println(result);
System.out.println("*** END ***");
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
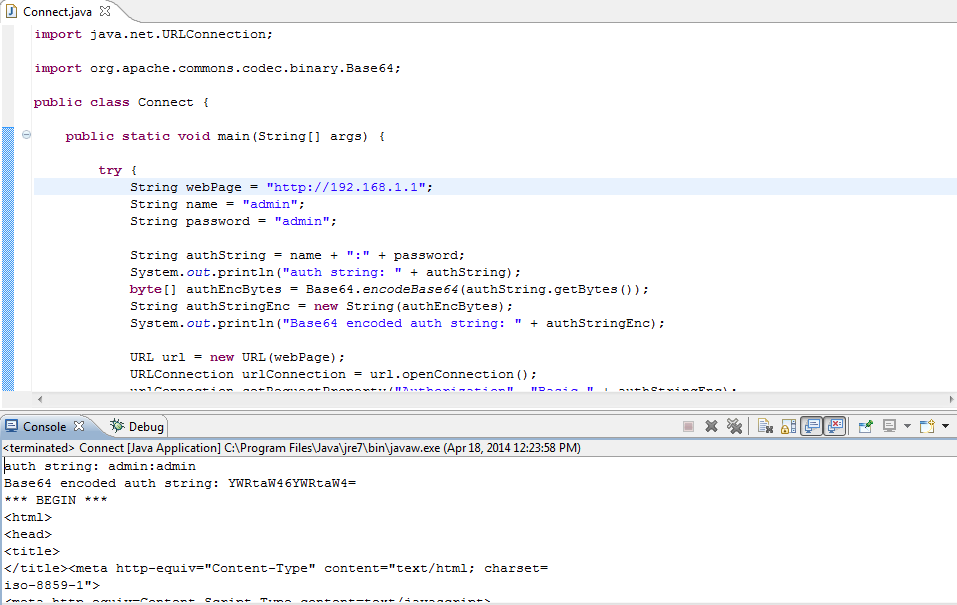
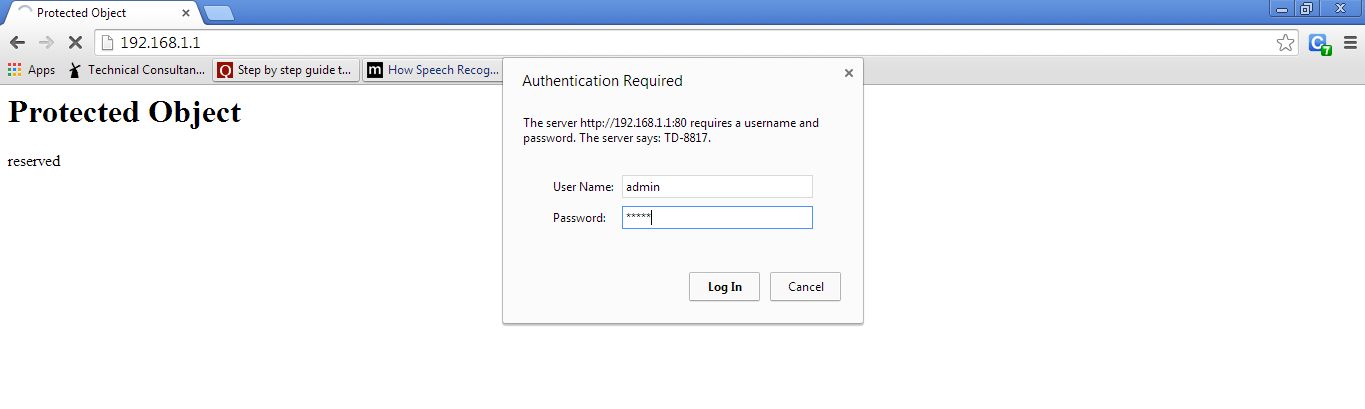
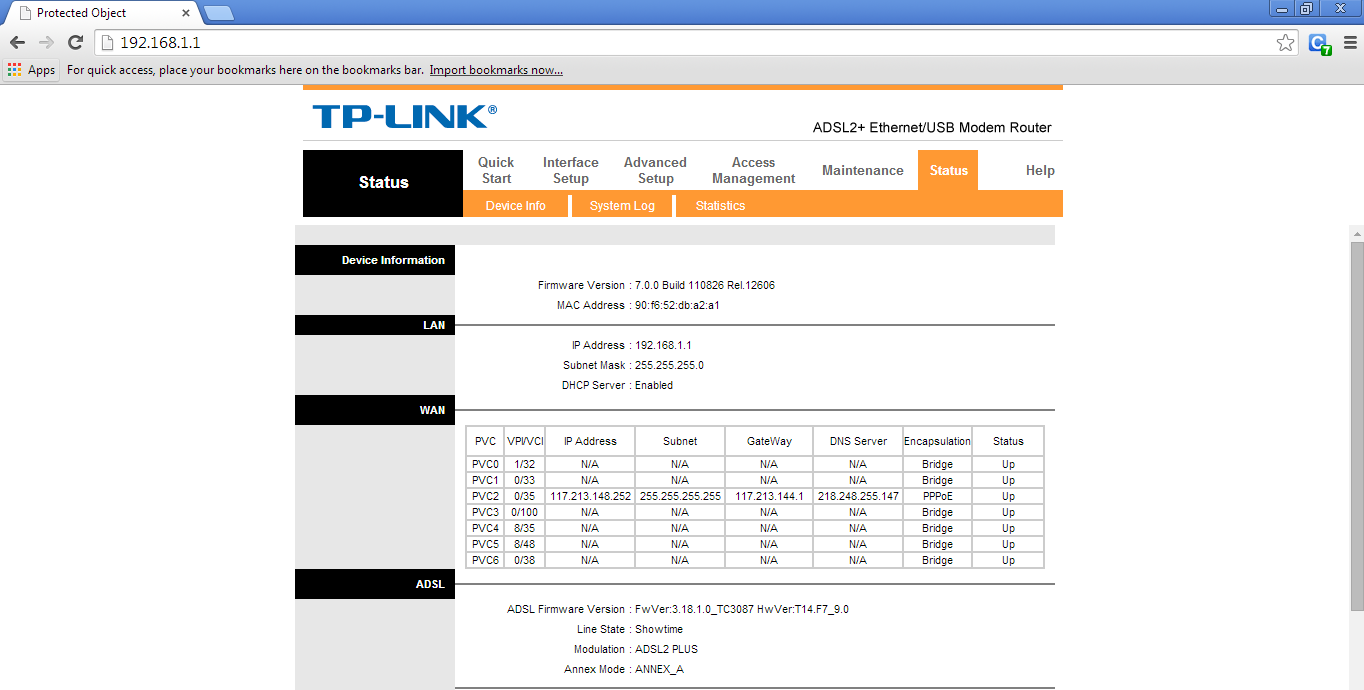
Code similar to Connect.java allows me to connect to my TP-LINK router and read the current IP address of my router. I can take this IP information and send it to a web application deployed on the web. As a result, I can then forward requests from the deployed web application through the router to my local machine. This technique requires me to forward requests to my router to port 8080 to my local machine on port 8080, as shown below.
No comments:
Post a Comment