- Constant pointer and pointer to constant.
- Pointer to pointer with an example
- Array of pointers with an example
- Pointer to functions with an example
1. C Constant Pointer and Pointer to Constant
As a developer, you should understand the difference between constant pointer and pointer to constant.
C Constant pointer
A pointer is said to be constant pointer when the address its pointing to cannot be changed.
Lets take an example :
char ch, c;char *ptr = &ch;ptr = &c;
In the above example we defined two characters (‘ch’ and ‘c’) and a character pointer ‘ptr’. First, the pointer ‘ptr’ contained the address of ‘ch’ and in the next line it contained the address of ‘c’. In other words, we can say that Initially ‘ptr’ pointed to ‘ch’ and then it pointed to ‘c’.
But in case of a constant pointer, once a pointer holds an address, it cannot change it. This means a constant pointer, if already pointing to an address, cannot point to a new address.
If we see the example above, then if ‘ptr’ would have been a constant pointer, then the third line would have not been valid.
A constant pointer is declared as :
<type-of-pointer> *const <name-of-pointer>
For example :
#include<stdio.h>int main(void) {char c = 'a';char ch = 'c';char *const ptr = &ch; // A constant pointerptr = &c; // Trying to assign new address to a constant pointer. WRONG!!!!return 0;
}
When the code above is compiled, compiler gives the following error :
$ gcc -Wall constptr.c -o constptrconstptr.c: In function ‘main’:of read-only variable ‘ptr’constptr.c:9: error: assignment
So we see that, as expected, compiler throws an error since we tried to change the address held by constant pointer.
Now, we should be clear with this concept. Lets move on.
C Pointer to Constant
This concept is easy to understand as the name simplifies the concept. Yes, as the name itself suggests, this type of pointer cannot change the value at the address pointed by it.
Lets understand this through an example :
char ch = 'c';char *ptr = &ch;*ptr = 'a';
In the above example, we used a character pointer ‘ptr’ that points to character ‘ch’. In the last line, we change the value at address pointer by ‘ptr’. But if this would have been a pointer to a constant, then the last line would have been invalid because a pointer to a constant cannot change the value at the address its pointing to.
A pointer to a constant is declared as :
const <type-of-pointer> *<name-of-pointer>;
For example :
#include<stdio.h>int main(void) {const char *p;char ch = 'c';
tr = &ch; // A constant pointer 'ptr' pointing to 'ch'*ptr = 'a';// WRONG!!! Cannot change the value at address pointed by 'ptr'.return 0;}
When the above code was compiled, compiler gave the following error :
$ gcc -Wall ptr2const.c -o ptr2constptr2const.c: In function ‘main’:of read-only location ‘*ptr’ptr2const.c:7: error: assignment
So now we know the reason behind the error above ie we cannot change the value pointed to by a constant pointer.
2. C Pointer to Pointer
Till now we have used or learned pointer to a data type like character, integer etc. But in this section we will learn about pointers pointing to pointers.
As the definition of pointer says that its a special variable that can store the address of an other variable. Then the other variable can very well be a pointer. This means that its perfectly legal for a pointer to be pointing to another pointer.
Lets suppose we have a pointer ‘p1′ that points to yet another pointer ‘p2′ that points to a character ‘ch’. In memory, the three variables can be visualized as :
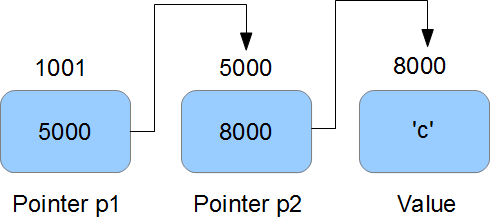
So we can see that in memory, pointer p1 holds the address of pointer p2. Pointer p2 holds the address of character ‘ch’.
So ‘p2′ is pointer to character ‘ch’, while ‘p1′ is pointer to ‘p2′ or we can also say that ‘p2′ is a pointer to pointer to character ‘ch’.
Now, in code ‘p2′ can be declared as :
char *p2 = &ch;
But ‘p1′ is declared as :
char **p1 = &p2;
So we see that ‘p1′ is a double pointer (ie pointer to a pointer to a character) and hence the two *s in declaration.
Now,
- ‘p1′ is the address of ‘p2′ ie 5000
- ‘*p1′ is the value held by ‘p2′ ie 8000
- ‘**p1′ is the value at 8000 ie ‘c’
I think that should pretty much clear the concept, lets take a small example :
#include<stdio.h>int main(void) {char *p = NULL;char **ptr = NULL; char c = 'd'; p = &c;
ptr = &p;
printf("\n c = [%c]n",c);
printf("\n **ptr = [%c]n",**ptr);
printf("\n *p = [%c]\n",*p);
return 0; }
Here is the output :
$ ./doubleptrc = [d]**ptr = [d]*p = [d]
3. C Array of Pointers
Just like array of integers or characters, there can be array of pointers too.
An array of pointers can be declared as :
<type> *<name>[<number-of-elements];
For example :
char *ptr[3];
The above line declares an array of three character pointers.
Lets take a working example :
#include<stdio.h>int main(void) {manshu"; char *p2 = "Achar *p1 = "H irora"; char *p3 = "India";1; arr[1] = p2char *arr[3]; arr[0] = p; arr[2] = p3;] \n",p1); printf("\n p2 = [%printf("\n p1 = [% ss] \n",p2); printf("\n p3 = [%s] \n",p3);tf("\n arr[1] = [%s] \n",arr[1]); priprintf("\n arr[0] = [%s] \n",arr[0]); pri nntf("\n arr[2] = [%s] \n",arr[2]); return 0;}
In the above code, we took three pointers pointing to three strings. Then we declared an array that can contain three pointers. We assigned the pointers ‘p1′, ‘p2′ and ‘p3′ to the 0,1 and 2 index of array. Let’s see the output :
$ ./arrayofptrp1 = [Himanshu]p2 = [Arora]rr[0] = [Himanp3 = [India] ashu] arr[1] = [Arora]arr[2] = [India]
So we see that array now holds the address of strings.
4. C Function Pointers
Just like pointer to characters, integers etc, we can have pointers to functions.
A function pointer can be declared as :
<return type of function> (*<name of pointer>) (type of function arguments)
For example :
int (*fptr)(int, int)
The above line declares a function pointer ‘fptr’ that can point to a function whose return type is ‘int’ and takes two integers as arguments.
Lets take a working example :
#include<stdio.h>int func (int a, int b){printf("\n a = %d\n",a);printf("\n b = %d\n",b);d) { int(return 0; } int main(void*fptr)(int,int); // Function pointernction pointer func(2,3); fptr(2,3); refptr = func; // Assign address to f uturn 0;}
In the above example, we defined a function ‘func’ that takes two integers as inputs and returns an integer. In the main() function, we declare a function pointer ‘fptr’ and then assign value to it. Note that, name of the function can be treated as starting address of the function so we can assign the address of function to function pointer using function’s name. Lets see the output :
$ ./fptra = 2a = 2b = 3 b = 3
So from the output we see that calling the function through function pointer produces the same output as calling the function from its name.